In the ever-evolving landscape of web development, two powerhouses, Redux Toolkit and TypeScript, emerge as game-changers. Redux Toolkit offers a streamlined and opinionated approach to state management in Redux applications, while TypeScript enhances your JavaScript codebase with the rigidity of static type checking. This amalgamation has the potential to create a development ecosystem that prioritizes maintainability and minimizes errors. In this insightful guide, we will steer you through the intricacies of diving into the world of Redux Toolkit when coupled with TypeScript.
Exploring Redux
Redux stands as a robust state management library for JavaScript applications, often employed alongside React. It functions as a centralized container for your application’s state, embracing a one-way data flow that ensures predictability. Through its architecture, Redux simplifies intricate state management scenarios and aids in the debugging process by offering a clear structure for tracking data changes over time.
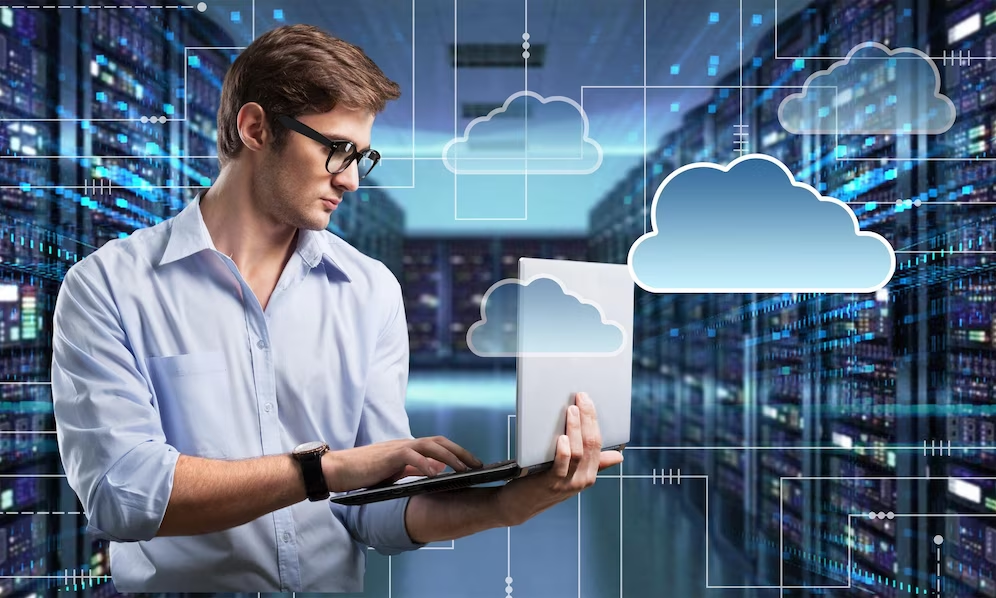
Introducing TypeScript
TypeScript emerges as a superset of JavaScript, infused with static typing capabilities. This extension empowers developers to assign types to variables, function parameters, and return values, enhancing code predictability and minimizing runtime errors. Embracing TypeScript equips the development process with error detection during coding, and the resulting code becomes more self-descriptive and comprehensible.
Rediscovering Redux Toolkit
Redux Toolkit emerges as an official package designed to streamline and refine various aspects of Redux implementation. Within its toolkit, developers uncover utilities that trim down boilerplate code and promote coding best practices. A notable inclusion is the createSlice function, offering a concise method for defining reducers and actions.
Moreover, Redux Toolkit introduces createAsyncThunk to simplify the management of asynchronous actions, mitigating the intricacies of handling network requests. By embracing Redux Toolkit, developers elevate productivity and cultivate the creation of maintainable Redux applications.
Unveiling the Synergy: Redux Toolkit and TypeScript
The synergy between Redux Toolkit and TypeScript forms a dynamic duo that revolutionizes modern web development. Redux Toolkit introduces a simplified yet robust approach to managing state in Redux applications, and TypeScript brings the promise of static type checking to your JavaScript codebase. The outcome? Enhanced code quality, improved error detection, and a smoother development experience. In this article, we embark on a journey to explore the harmonious blend of Redux Toolkit and TypeScript and how it can empower you as a developer.
Building the Foundation: Introducing Redux Toolkit and TypeScript
Picture a foundation of a web development project where the pillars of Redux Toolkit and TypeScript stand tall. Redux Toolkit, renowned for its ability to declutter state management, finds its match in TypeScript’s ability to enforce type safety. As these technologies converge, they empower developers to create applications that are not only robust but also maintainable. This guide is your gateway to unlocking the potential of this powerful duo. We’ll walk you through the fusion of Redux Toolkit and TypeScript, equipping you with the skills to create modern web applications with a focus on structure and reliability.
Prerequisites
Before you dive headfirst into the dynamic world of Redux Toolkit and TypeScript integration, it’s vital to lay down a robust groundwork. Familiarity with essential technologies like React, Redux, and TypeScript is akin to having a well-tuned compass that guides your way. These foundational tools will provide the canvas upon which you’ll create the intricate artwork of your Redux Toolkit and TypeScript fusion.
Additionally, ensuring your development environment is primed for action is paramount. The installation of Node.js and npm (Node Package Manager) on your machine serves as the ignition key for your development engine. With these tools at your disposal, you can effortlessly install dependencies and execute vital scripts, propelling your project forward.
By building on your existing knowledge and cultivating an environment ripe for innovation, you’re ready to embark on the exhilarating journey of Redux Toolkit and TypeScript synergy. Prepare to unravel new horizons of efficient state management and robust code with a blend of these potent technologies.
Step 1: Creating a New React Application
To begin, let’s create a new React application. Open your terminal and run the following command:
npx create-react-app my-redux-app –template typescript-react-app my-redux-app –template
This command will create a new React application with TypeScript configuration.
Step 2: Installing Redux Toolkit
Navigate to your project directory using the terminal and install Redux Toolkit and React Redux by running:
npm install @reduxjs/toolkit react-reduxact-redux
Step 3: Setting Up the Store
In Redux Toolkit, the store setup is greatly simplified. Create a new file called store.ts in the src folder of your project. Here’s a basic setup:
import { configureStore } from ‘@reduxjs/toolkit’; const store = configureStore({reducer: { // Add your reducers here }, }); export default store;
Step 4: Creating a Slice
Slices in Redux Toolkit are used to define parts of your Redux store. Create a new file called counterSlice.ts in the src folder. Here’s an example of a counter slice:
import { createSlice } from ‘@reduxjs/toolkit’; interface CounterState { value: number; }const initialState: CounterState = { value: 0, }; const counterSlice = createSlice({name: ‘counter’, initialState, reducers: { increment: (state) => { state.value += 1; },decrement: (state) => { state.value -= 1; }, }, }); export const { increment, decrement } = counterSlice.actions; export default counterSlice.reducer;
Step 5: Connecting Redux to Your App
Open the src/index.tsx file and import the necessary modules:
import React from ‘react’; import ReactDOM from ‘react-dom’; import { Provider } from’react-redux’; import store from ‘./store’; import App from ‘./App’; ReactDOM.render(<Provider store={store}> <App /> </Provider>, document.getElementById(‘root’) );
Step 6: Using Redux State in Components
You can now use Redux state in your components. Import the necessary modules and use the useSelector and useDispatch hooks:
import React from ‘react’; import { useSelector, useDispatch } from ‘react-redux’; import{ increment, decrement } from ‘./counterSlice’; function App() { const counter = useSelector((state) => state.counter.value); const dispatch = useDispatch(); return (<div> <h1>Counter: {counter}</h1> <button onClick={() =>dispatch(increment())}>Increment</button> <button onClick={() =>dispatch(decrement())}>Decrement</button> </div> ); } export default App;
Conclusion
In conclusion, the marriage of Redux Toolkit and TypeScript emerges as a potent elixir in the realm of web development. The amalgamation of Redux Toolkit’s elegant streamlining and TypeScript’s vigilant type enforcement establishes a formidable duo that empowers developers to craft robust, error-resilient React applications.
As you traverse the intricacies of these technologies, you’ll unearth a development experience that marries simplicity and precision. Redux Toolkit’s orchestration of state management intricacies, combined with TypeScript’s watchful gaze over types, forges a path towards codebases that are both reliable and scalable.
With Redux Toolkit’s hassle-free configuration and TypeScript’s unwavering type checks at your disposal, you’ll navigate the ever-evolving landscape of web development with a heightened sense of confidence and proficiency. Your React applications will not only thrive in the present but will also be fortified for future challenges.
So, seize this opportunity to embrace Redux Toolkit and TypeScript. Immerse yourself in learning, experimentation, and innovation as you embark on a journey that promises to revolutionize your development process. Embrace the elegance of Redux Toolkit and the robustness of TypeScript, and unveil a new era of excellence in your web development endeavors.
Frequently Asked Questions
Combining Redux Toolkit’s streamlined state management and TypeScript’s static type checking offers a powerful duo. The dynamic nature of JavaScript is enhanced by TypeScript’s type safety, making your code more reliable and maintainable. This collaboration can lead to more efficient development and fewer runtime errors.
While familiarity with Redux and TypeScript can be advantageous, this guide caters to beginners and those already acquainted with these technologies. A grasp of React fundamentals, the foundation of Redux, and basic TypeScript concepts will facilitate understanding.
Redux Toolkit simplifies many Redux tasks, such as reducing boilerplate and handling immutability. For TypeScript users, it aligns with static typing principles and enhances code predictability. This results in a smoother development experience and more robust applications.
The article provides a comprehensive walkthrough for project setup with Redux Toolkit and TypeScript. It encompasses installing dependencies, configuring the Redux store, and harnessing Redux Toolkit’s features like createSlice and createAsyncThunk.
Certainly! Integrating Redux Toolkit and TypeScript into existing projects involves adapting your Redux setup to Redux Toolkit’s utilities. Migrating existing Redux code to utilize Redux Toolkit’s syntax is a gradual process that can enhance your codebase.
While the rewards of Redux Toolkit and TypeScript are significant, acclimatizing to new conventions and syntax might pose an initial challenge. However, investing time in mastering these tools pays off in terms of code quality, fewer bugs, and long-term development efficiency.
While this article provides a comprehensive starting point, further exploration is encouraged. The official documentation for both Redux Toolkit and TypeScript offers in-depth insights. Online tutorials, forums, and communities are valuable resources for expanding your knowledge.
Absolutely! Redux Toolkit and TypeScript are versatile companions for various web development ecosystems. They integrate seamlessly with other libraries and frameworks, ensuring your state management and code quality remain robust across diverse environments.
Indeed, Redux Toolkit caters to projects of various magnitudes. Its simplicity and built-in tools benefit both smaller and larger applications. For small projects, it expedites state management, while in larger ventures, it streamlines complex scenarios.
Initially, there might be a slight learning curve due to Redux Toolkit’s syntax and TypeScript’s type declarations. However, the early error detection and code predictability provided by TypeScript and the streamlined Redux development process will likely yield time savings in the long run.